python 爬虫入门到进阶(五)
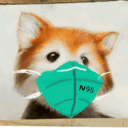
实战项目妹子图爬取
多图预警
就不放太多了,等下可以自己尝试一下
打开我们要爬去的网站,f12 打开控制台
导入头
1 | # -*- coding: utf-8 -*- |
设置编码和头
1 | sys.setdefaultencoding('utf8') |
定义存储路径
1 | path = "/home/gwj/snap/demo/image/" |
解析获取的页面
1 | soup = BeautifulSoup(respons.read(),"lxml") |
这里打印出 a 标签,lxml 是解析器,respons.read () 存储页面的内容
由于我们要进入到每个子页面才能保存图片,这里我们要先找到最大页码,以此决定循环次数(每次打开子页面,保存图片是一个循环)
1 | #最大页数在span标签中的第10个 |
解释一下 soup.find()
查找某一个,soup.find_all
查找所有的,返回一个列表
soup.find‘img’ 查找 img 的 src 链接属性
class_ 获取目标的类名
不同页面之间都有相似的地方
这里存储一下这个 url,用来构建访问子页面的链接
1 | same_url = 'http://www.mzitu.com/page/' |
循环访问子页面 格式为 page+n
1 | for n in range(1,int(max_page)+1): |
访问详情页面,提取用户名,URL
1 | #提取用户名 |
上方第 18 行代码,判断数据长度是否为空,这里是记录单个妹子的图片最大页数,同时本页包含了图片的 url
第 12 行代码,创建 path + 文件名的新目录
22 行,判断目录是否 已经创建,如果已经创建,则跳出本次循环
如有不理解可在评论区评论
获取图片链接
1 | for num in range(1,int(pic_max)+1): |
判断链接是否为空(防止报错)
1 | if pic_url is None: |
截取链接'/
后的字符作为文件名,写入文件
1 | file_name = pic_url['src'].split(r'/')[-1] |
完整代码
1 | # -*- coding: utf-8 -*- |
第一次写不太完善,有问题评论
下一节:另一种方式爬取妹子图
表情包获取
- 标题: python 爬虫入门到进阶(五)
- 作者: tsvico
- 创建于 : 2018-06-22 16:06:30
- 更新于 : 2021-03-06 20:21:07
- 链接: https://blog.tbox.fun/2018/81e23d33.html
- 版权声明: 本文章采用 CC BY-NC-SA 4.0 进行许可。
评论